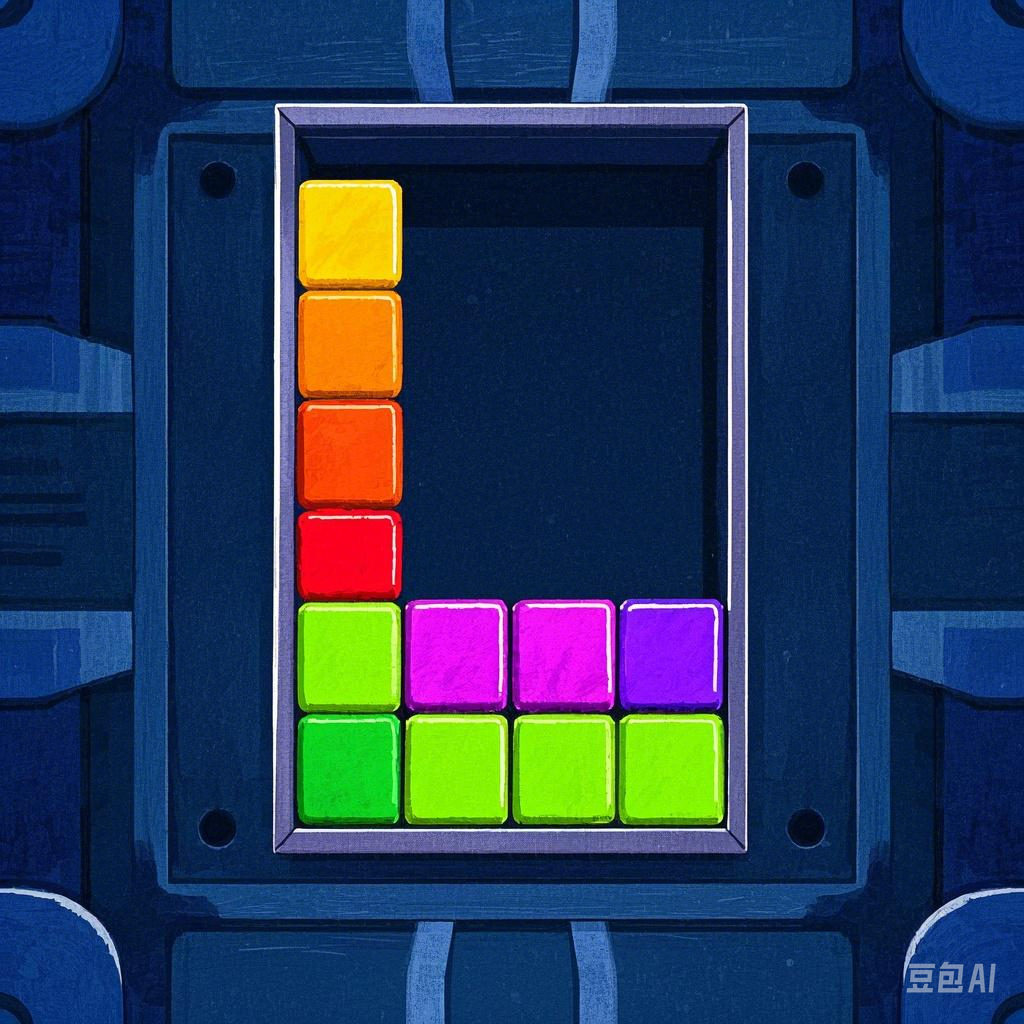
AI编程挑战: 一句提示实现俄罗斯方块游戏程序
豆包, Kimi, 百度, GPT 测试
上次完成了一句提示实现贪吃蛇游戏程序后, 这次我们决定以俄罗斯方块游戏作为测试用例。
本次测试与上次的区别是:
- 游戏复杂度增加了;
- 通过多轮对话提高代码质量, 以减少误差。
候选 AI 模型:
豆包 AI, 免费网页版
Kimi AI, 免费网页版
百度 文心一言, 免费网页版
GPT-4o, ambot.chat 网页版
测试提示词:
准备提示:你知道俄罗斯方块的游戏吗
正题提示:请给我生成一个网页版的俄罗斯方块游戏。用i, j, k, l 控制动作, 生成单个文件的html文件。
递归提示: (根据上一轮答复的内容和代码运行结果, 作出递归提示, 驱动AI 模型修正答复结果, 以获得更好的代码质量。 递归提示不超过三轮。 )
附加提示:(在递归提示完成前, 如果代码能够正常运行, 则进行不超过三轮的附加提示。 ) 代码可以正常运行。 现在需要增加以下这些功能:1. 一轮游戏结束以后, 需要弹出一个游戏结束的提示。用户确认以后, 开始新的一轮游戏。2. 在游戏框外面, 提示下一个出现的方块。
运行环境
操作系统 MacOS
浏览器 谷歌 Chrome, 微软 Edge
开发环境 MS VS code 免费版
测试分项
网页加载正确
- html文件在浏览器中能正常打开。
响应控制正确
- 出现正确的多种方块, 自由下落, 对控制键有响应。
代码逻辑正确
- 方块触底判断正确, 方块堆叠正确, 方块触顶判断正确, 游戏结束判断正确。
附加功能正确
- 实现游戏结束提示互动, 循环游戏, 出现方块提示
测试结果
豆包 AI
- 网页加载正确, 响应控制正确, 代码逻辑错误, 附加功能错误。
不能判断方块触底, 方块堆叠在游戏框外面。 方块提示错误。
Kimi AI
- 网页加载正确, 响应控制错误, 代码逻辑错误, 附加功能错误。
代码运行后出现黑色游戏框, 无操控响应。
百度 AI
- 网页加载正确, 响应控制错误, 代码逻辑正确, 附加功能错误。
百度 AI 似乎不能正确实现多轮对话, 每一轮的递归提示都会得到不同结果, 为百度额外增加三轮提示。
GPT-4o ( ambot.chat)
- 网页加载正确, 响应控制正确, 代码逻辑正确, 附加功能正确。
在递归提示中, GPT自动想到要为方块增加颜色。
结论:
在一句提示实现俄罗斯方块游戏程序的测试中, GPT-4o完胜。
附 GPT-4o 生产的完整代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>俄罗斯方块</title>
<style>
body { display: flex; flex-direction: column; justify-content: center; align-items: center; height: 100vh; background-color: #f0f0f0; }
#next { margin-bottom: 20px; }
canvas { background-color: #000; }
</style>
</head>
<body>
<canvas id="next" width="120" height="120"></canvas>
<canvas id="tetris" width="240" height="400"></canvas>
<script>
const canvas = document.getElementById('tetris');
const context = canvas.getContext('2d');
context.scale(20, 20);
const nextCanvas = document.getElementById('next');
const nextContext = nextCanvas.getContext('2d');
nextContext.scale(20, 20);
function createMatrix(w, h) {
const matrix = [];
while (h--) {
matrix.push(new Array(w).fill(0));
}
return matrix;
}
function createPiece(type) {
if (type === 'T') {
return [
[0, 1, 0],
[1, 1, 1],
[0, 0, 0],
];
} else if (type === 'O') {
return [
[2, 2],
[2, 2],
];
} else if (type === 'L') {
return [
[0, 3, 0],
[0, 3, 0],
[0, 3, 3],
];
} else if (type === 'J') {
return [
[0, 4, 0],
[0, 4, 0],
[4, 4, 0],
];
} else if (type === 'I') {
return [
[0, 5, 0, 0],
[0, 5, 0, 0],
[0, 5, 0, 0],
[0, 5, 0, 0],
];
} else if (type === 'S') {
return [
[0, 6, 6],
[6, 6, 0],
[0, 0, 0],
];
} else if (type === 'Z') {
return [
[7, 7, 0],
[0, 7, 7],
[0, 0, 0],
];
}
}
function drawMatrix(matrix, offset, ctx) {
const colors = [
null,
'red',
'yellow',
'orange',
'blue',
'cyan',
'green',
'purple'
];
matrix.forEach((row, y) => {
row.forEach((value, x) => {
if (value !== 0) {
ctx.fillStyle = colors[value];
ctx.fillRect(x + offset.x, y + offset.y, 1, 1);
}
});
});
}
function draw() {
context.clearRect(0, 0, canvas.width, canvas.height);
drawMatrix(arena, {x: 0, y: 0}, context);
drawMatrix(player.matrix, player.pos, context);
nextContext.clearRect(0, 0, nextCanvas.width, nextCanvas.height);
drawMatrix(nextPiece, {x: 1, y: 1}, nextContext);
}
function merge(arena, player) {
player.matrix.forEach((row, y) => {
row.forEach((value, x) => {
if (value !== 0) {
arena[y + player.pos.y][x + player.pos.x] = value;
}
});
});
}
function collide(arena, player) {
const [m, o] = [player.matrix, player.pos];
for (let y = 0; y < m.length; ++y) {
for (let x = 0; x < m[y].length; ++x) {
if (m[y][x] !== 0 &&
(arena[y + o.y] &&
arena[y + o.y][x + o.x]) !== 0) {
return true;
}
}
}
return false;
}
function rotate(matrix, dir) {
for (let y = 0; y < matrix.length; ++y) {
for (let x = 0; x < y; ++x) {
[
matrix[x][y],
matrix[y][x],
] = [
matrix[y][x],
matrix[x][y],
];
}
}
if (dir > 0) {
matrix.forEach(row => row.reverse());
} else {
matrix.reverse();
}
}
function playerDrop() {
player.pos.y++;
if (collide(arena, player)) {
player.pos.y--;
merge(arena, player);
resetPlayer();
arenaSweep();
if (collide(arena, player)) {
endGame();
}
}
dropCounter = 0;
}
function playerMove(dir) {
player.pos.x += dir;
if (collide(arena, player)) {
player.pos.x -= dir;
}
}
function resetPlayer() {
player.matrix = nextPiece;
player.pos.y = 0;
player.pos.x = (arena[0].length / 2 | 0) - (player.matrix[0].length / 2 | 0);
nextPiece = createPiece(pieces[Math.floor(Math.random() * pieces.length)]);
}
function arenaSweep() {
outer: for (let y = arena.length - 1; y > 0; --y) {
for (let x = 0; x < arena[y].length; ++x) {
if (arena[y][x] === 0) {
continue outer;
}
}
const row = arena.splice(y, 1)[0].fill(0);
arena.unshift(row);
++y;
}
}
function endGame() {
cancelAnimationFrame(animationId);
alert('游戏结束');
startGame();
}
function startGame() {
arena.forEach(row => row.fill(0));
resetPlayer();
update();
}
const arena = createMatrix(12, 20);
let dropCounter = 0;
let dropInterval = 1000;
let lastTime = 0;
let animationId;
const pieces = 'IJLOSTZ';
let nextPiece = createPiece(pieces[Math.floor(Math.random() * pieces.length)]);
function update(time = 0) {
const deltaTime = time - lastTime;
lastTime = time;
dropCounter += deltaTime;
if (dropCounter > dropInterval) {
playerDrop();
}
draw();
animationId = requestAnimationFrame(update);
}
const player = {
pos: {x: 0, y: 0},
matrix: null,
};
document.addEventListener('keydown', event => {
if (event.key === 'j') {
playerMove(-1);
} else if (event.key === 'l') {
playerMove(1);
} else if (event.key === 'k') {
playerDrop();
} else if (event.key === 'i') {
rotate(player.matrix, 1);
}
});
startGame();
</script>
</body>
</html>
更多推荐
所有评论(0)